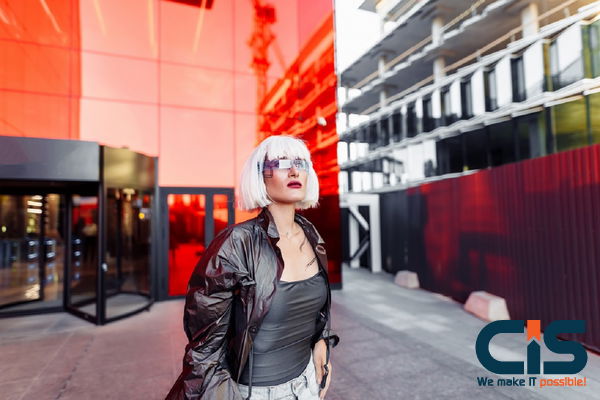
By 2030, the global developer community is expected to surpass 45 million, but one debate still continues, what's the best way to write code?
When building apps, websites, or software, two popular styles are used: imperative programming and declarative programming. These styles help you tell a computer what to do, but in very different ways.
One style gives step-by-step directions, like a recipe. The other tells the end goal and lets the computer figure out the steps.
Both are useful. But knowing when to use each one can save time, lower errors, and make your code easier to manage.
In this blog, we'll break it all down with simple words, real-life code examples, and tips to choose the right approach for your next project.
What Is Imperative Programming?
Imperative programming is a way of writing code that tells the computer exactly how to do things. It works by giving step-by-step instructions to the system, explaining each action it must take. This approach relies on control flow elements like loops, conditionals, and variable assignments to manage how the program runs.
Popular languages such as Java, C, and Python use this style because they allow developers to control every detail of the process. Think of imperative programming like following a recipe: you chop the ingredients, heat the pan, and add everything in order to create the dish.
Imperative code focuses on the "how," the explicit steps needed to complete a task. On the other hand, declarative programming tells the system "what" outcome you want without specifying the steps.
While imperative programming gives you fine-grained control, declarative styles can simplify code and improve readability.
In software development, the debate between imperative programming and declarative programming continues as both have their advantages. Knowing when to use each can improve your coding efficiency and software quality.
Imperative languages are great for tasks needing precise control, while declarative languages excel in expressing desired results with less code.
What Is Declarative Programming?
Declarative programming emphasizes the desired result over the means of achieving it. Instead of giving the computer detailed steps, you describe the desired result, and the system figures out the process.
Code written in this form is clearer and easier to read because the control flow is hidden. It helps reduce errors since programmers don't have to manage every small detail.
Common examples include SQL, which lets you request data without specifying how to fetch it, HTML, which defines webpage layouts, and React, a popular tool for building user interfaces by declaring what the UI should look like.
Think of declarative programming like ordering food at a restaurant. You tell the waiter what you want, not how to prepare it. The kitchen handles the details behind the scenes.
Understanding imperative and declarative programming helps you choose the right style for your projects. While imperative programming controls every step, declarative programming focuses on clarity and simplicity.
Understanding imperative programming vs declarative programming is key to using each approach effectively, depending on your project's needs.
Key Differences: Imperative vs Declarative Programming
The main difference between imperative vs declarative programming is control versus abstraction. Imperative programming tells the computer exactly how to do a task, step by step. Declarative programming allows the system to take care of the specifics while concentrating on the desired outcome.
Here are some key differences:
Control vs Abstraction:
Imperative programming controls every step. Declarative programming concentrates on the intended result while abstracting the method.
Explicit Logic vs Intent-Driven Design:
Imperative code writes out the full logic flow. Declarative code states the intent without specifying the process.
Readability:
Declarative code is usually easier to read because it hides complexity. Imperative code can be more detailed and harder to follow.
Learning Curve:
Beginners often find declarative and imperative programming easier since they focus on what to do. Imperative programming requires understanding how to do each step.
Performance:
Imperative programming often allows more optimization since you control every action. Declarative programming relies on the system's efficiency.
Real-world examples highlight these differences well. SQL is declarative, asking for data without describing how to find it. Writing data processing in Java is imperative, managing each operation step by step.
Understanding imperative programming vs declarative programming helps you pick the right style for your project. Choosing the right paradigm, imperative vs declarative programming, can speed up development and improve code quality.
Read More: Top Programming Languages for Machine Learning: A Complete Guide
Code Examples: See the Difference in Action
To truly understand imperative and declarative programming, seeing real examples helps a lot. Let's walk through two common scenarios where both styles are used.
Example 1: Filtering Even Numbers in Python
In the imperative programming style, you give the computer a set of clear instructions. Imagine you have a list of numbers. You start by creating an empty list that will hold even numbers.Then, you write a loop that goes through each number in the original list one by one. Inside that loop, you check if a number is even by testing if it divides evenly by 2. You include that number in the updated list if it is. Finally, you print the list of even numbers. Here, you control every single step of the process.
On the other hand, declarative programming focuses on what you want to achieve, not how to do it. Using Python's built-in filter function, you just specify a rule, keep only numbers that are even. The computer then handles the details of applying that rule to the list. This results in shorter, cleaner code that's easier to read and maintain.
Example 2: Querying Data
When working with data, the difference becomes even clearer. In an imperative approach, you might write code to manually scan through each record in a dataset. For every user, you check if their age is greater than 30. The user gets added to a new list if such is the case. This method gives you total control but can be long and complex.
In contrast, the declarative approach uses a language like SQL. You write a simple query that says, "Select all users where age is over 30." You don't explain how to loop through data or check each record. The database takes care of those details for you, making your code easier to write and understand.
Why This Matters
These examples highlight the core difference in imperative an ddeclarative programming language styles. Imperative code spells out every step and keeps tight control. Declarative code describes what outcome you want and lets the system handle the rest.
Choosing between these styles depends on your project needs. If you want detailed control and optimization, imperative might be the way to go. If you want simple, readable code that's easy to maintain, declarative is often better.
Choosing Between Imperative and Declarative Programming
Choosing between imperative and declarative programming depends on your project's needs. Each style has strengths that fit different situations.
Use imperative programming when performance matters most. This approach gives you precise control over every step, which is great for tasks like game development, embedded systems, or anything requiring tight optimization. It lets you manage how the program runs, which can improve speed and resource use.
On the other hand, declarative programming shines when you want fast development and easier maintenance. It lets you focus on what the program should do instead of how to do it. This style works well for user interfaces, database queries, and configuration tasks. It reduces code complexity and makes it easier for teams to understand and update the code.
Most modern projects use a mix. Combining declarative vs imperative programming lets you get the best of both worlds. You can write simple, clear code where possible and switch to imperative code when you need more control.
Understanding when to use each style is key to building efficient, maintainable software. If you want advice on choosing the right approach or blending these styles, we're here to help with your next project.
Read Also: Trending Top 150 Programming Languages
Pros and Cons: A Balanced Perspective
When comparing imperative vs declarative programming, it's important to understand the strengths and weaknesses of each approach. Here's a clear breakdown:
Imperative Programming Pros
- Gives precise control over every step of the process
- Allows for detailed optimization and fine-tuning
- Ideal for performance-critical applications
Imperative Programming Cons
- Requires writing more code, which can be verbose
- can eventually get more intricate and challenging to manage.
- Demands careful management to avoid bugs
Declarative Programming Pros
- Produces cleaner and more readable code
- Speeds up development by focusing on what needs to be done, not how
- Easier to maintain due to higher abstraction levels
Declarative Programming Cons
- Offers less control over the exact execution details
- Requires a mindset shift, especially for developers used to step-by-step coding
- Can sometimes hide performance costs under the hood
Understanding these points helps when choosing between imperative programming and declarative programming. Each approach has its place, depending on the project's needs.
Many modern applications use a mix of both styles to get the best of control, clarity, and speed.
How Modern Frameworks Combine Both Paradigms
Today's popular frameworks like React, Angular, and Vue blend imperative and declarative programming styles to deliver powerful and flexible apps. These tools use declarative syntax to describe what the user interface should look like. At the same time, they rely on imperative logic to handle events, update states, and manage lifecycles behind the scenes.
This mix gives developers the best of both worlds. Declarative code makes UI easier to read and maintain. Meanwhile, imperative parts let programmers control how the app responds to user actions in detail. This combination improves developer productivity, reduces bugs, and helps apps run faster.
In our IT services work, we often use this hybrid approach for client projects. For example, with React, we write declarative components for clear layouts. Then, we add imperative event handlers to control animations or data updates. This strategy speeds up development cycles and leads to higher-quality software.
Many clients report better app performance and easier maintenance after switching to frameworks that balance declarative vs imperative programming. It shows how understanding imperative programming and declarative programming helps build modern, scalable software.If you want to explore how this blend of styles can improve your next project, we're here to guide you every step of the way
Conclusion
Knowing the difference between imperative and declarative programming helps developers write better, cleaner code. We've covered their definitions, key contrasts, and practical uses. Choosing the right paradigm improves performance and maintainability.
Mastering both gives you the flexibility to build future-proof software. At CISIN, we use this knowledge to create scalable, efficient solutions for our clients.
Ready to use professional assistance to advance your development projects? Contact CISIN today and let's build something great together.
Frequently Asked Questions (FAQs)
What are common imperative programming languages?
Imperative programming languages include Java, C, and Python. They focus on giving detailed step-by-step instructions, allowing developers to control exactly how the program runs. This approach works well for complex logic and performance needs. It remains a popular choice for many traditional software projects because of its precision and control.
Can declarative programming improve security?
Declarative programming can improve security by reducing errors and simplifying code. It hides complex control flows, making code easier to review and less prone to bugs. Fewer bugs mean fewer vulnerabilities for attackers to exploit. This style promotes writing cleaner, more reliable code and is often used in database queries and configuration management.
Is declarative programming harder to learn?
Declarative programming can be harder to learn because it requires a shift in thinking. Instead of telling the computer how to do tasks, you describe what you want. This may feel less intuitive for those used to step-by-step instructions. However, once learned, it helps developers write simpler and easier-to-maintain code. It's an important skill for working with many modern tools.
How does the paradigm affect debugging?
Debugging imperative code is often easier since you follow the program step by step. You can see exactly what happens at each line, which helps find errors. Declarative code is more abstract, so tracing bugs can be harder. However, declarative programs usually have fewer bugs to start with. Choosing the right style can make debugging smoother, depending on the task.
Should you mix imperative and declarative styles?
Mixing imperative and declarative styles is common and practical in modern development. Frameworks like React use declarative syntax alongside imperative logic to handle events and lifecycle tasks. This blend boosts productivity and keeps code clean and maintainable. It gives developers both control and simplicity. Using both paradigms helps build flexible, scalable apps.
Ready to Elevate Your Software Development?
Partner with CISIN to harness the power of both imperative and declarative programming styles. Our expert team helps you build clean, efficient, and scalable solutions tailored to your needs. Whether you want to optimize performance or speed up development, CISIN provides the guidance and support to bring your projects to life. Let's work together to create future-proof code that drives your success. Contact CISIN today to get started!