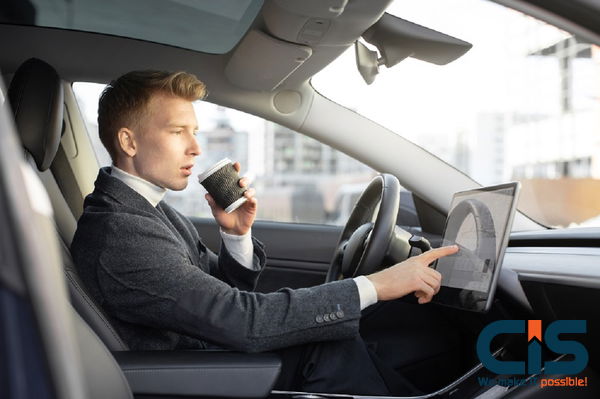
Choosing the right typing strategy isn't just a technical decision. It's the foundation for writing safer, faster, and more scalable code.
In programming, "typing" refers to how a language defines and manages the types of data, like numbers, text, or lists, that a program uses. There are two main approaches: static typing and dynamic typing. Static typing checks data types before the program runs, while dynamic typing checks them during execution.
Your choice between static and dynamic typing can significantly impact your code's safety, speed, and maintainability.
In this blog, we'll explore these differences and help you understand how to choose the right approach for your project.
What Is Static Typing?
Static typing is when a programming language requires you to define the type of data a variable holds, like numbers, words, or lists, before the code runs. These types are checked by the compiler during what's called compile-time.
If something doesn't match, the program won't run until you fix it.
Real-World Examples
Languages like Java, C++, and Rust use static typing. These languages are trusted in serious, large-scale systems, think banking platforms, healthcare apps, aerospace software, and enterprise tools. Why? Because catching errors early prevents big problems later.
The compiler catches this mistake before the program even starts. That means fewer runtime crashes and more reliable software.
How Compile-Time Type Checking Helps
When your code is checked before it runs, it's much easier to spot issues right away. This prevents common bugs, like passing text where numbers are expected or calling a function with the wrong input.
You fix problems while coding, not after your users find them.
Better for Big Projects
In small projects, these checks might not seem like a big deal. But in large codebases with many developers, static typing adds structure. It helps you:
- Understand what each variable is supposed to do
- Refactor code safely without breaking other parts
- Find and fix bugs faster
- Improve long-term maintenance
Static typing acts like a safety net. It keeps things consistent and easier to manage as the code grows.
Read More: Revolutionize Your Software Development: Automated Code Refactoring
What Is Dynamic Typing?
Dynamic typing means you don't have to tell the program what kind of data a variable holds before running the code. Instead, the program figures it out as it runs, a process called run-time type checking. This gives programmers more freedom to write code quickly without worrying about strict type rules upfront.
Real-World Examples
Languages like Python, JavaScript, and Ruby use dynamic typing. These languages are favorites for web development, scripting, and fast-moving projects where speed matters more than strict rules. Startups and agile teams often pick them because they speed up coding and make it easier to change things on the fly.
In this case, Python doesn't complain about changing the variable's type. But if your program tries to use data as a number later, it might cause errors.
How Run-Time Type Checking Works
Because dynamic typing checks data types while the program runs, some errors only appear during execution. This means:
- Writing code is faster without upfront type definitions
- You can quickly try out new ideas and change variables freely
- However, some bugs can sneak in and only show up when users run the program
This trade-off offers flexibility but needs careful testing to catch problems early.
Why Dynamic Typing Fits Agile and Startups
Dynamic typing shines when teams need to build and adjust features fast. Startups and agile developers love it because:
- They can prototype quickly without strict type rules
- It reduces time spent on setup and boilerplate code
- They can adapt to customer feedback and change the code easily
For example, a startup we worked with used JavaScript and Node.js to launch their app in just 10 days. Dynamic typing lets them focus on delivering features instead of worrying about types.
Code Safety: Static vs Dynamic Typing
When you're building software, whether it's a mobile app, a website, or a complex backend system, one of the most important choices you'll make is how your code handles data types.
Let's break down how each approach affects error detection, code reliability, and overall safety.
Error Detection: Catch Bugs Early or Face Runtime Surprises
Static typing checks your code before it runs. This means the compiler spots type mistakes early, before your users ever see a problem. For example, if you try to add a number and text, the compiler will stop you. This early catch prevents many common bugs and crashes.
On the other hand, dynamic typing waits until your program is running to check types. This flexibility is helpful but risky. If a variable changes type unexpectedly, your program might crash during use. These runtime surprises can be tricky to find and fix, especially in large or complex applications.
Impact on Code Reliability: The Real-World Risks of Dynamic Typing
Without safeguards, dynamic typing can introduce hidden bugs that show up later. For instance, when multiple developers work on a project, it's easier to accidentally pass the wrong data type to a function. This can cause errors that are hard to debug and can affect user experience or system stability.
Static typing creates a safety net. It forces everyone on the team to follow clear rules about data types. This leads to more consistent code and fewer unexpected failures. For critical systems, ike financial software or healthcare apps, this reliability is essential.
Tools Enhancing Dynamic Typing Safety: Bridging the Gap
The good news is that dynamic typing doesn't have to mean unsafe code. Tools like TypeScript for JavaScript and MyPy for Python add optional static type checking. These tools analyze your code and catch type errors before runtime, blending flexibility with safety.
Using these static analysis tools can help teams move fast while avoiding many common bugs. They act as a middle ground, giving you the benefits of dynamic typing with extra protection.
Choosing the right typing approach depends on your project's needs. Static typing is ideal for long-term stability and safety. Dynamic typing offers speed and flexibility, especially when paired with tools that boost code safety.
How Typing Impacts Code Speed and Performance
When building high-performing applications, speed matters. How a programming language handles typing, whether static or dynamic, can significantly impact how fast your code runs.
Compilation vs Interpretation: What It Means for Speed
One of the biggest performance differences between statically and dynamically typed languages comes down to how they run your code.
Statically typed languages, like Java and C++, use compilation. The source code is translated into machine-level instructions before execution.
This step allows the compiler to analyze the code in depth and apply performance optimizations, like inlining, loop unrolling, and memory management tweaks, before the program even starts.
Dynamically typed languages, like Python and JavaScript, use interpretation. This means the program is executed line by line at runtime.
Each variable's type is checked while the code runs, which adds overhead. That overhead may seem small in short scripts, but it adds up fast in data-heavy or CPU-intensive applications.
How Static Typing Gives You Performance Gains
Static typing allows for stronger, more predictable optimizations. Here's why:
- Known types mean faster execution: When the compiler knows what type a variable holds, it can allocate memory and choose the best machine instructions ahead of time.
- No type guessing at runtime: Static code avoids the extra logic required to figure out data types on the fly. This keeps performance tight and predictable.
- Better support for advanced compilers: Languages like Java and Rust benefit from Just-In-Time (JIT) and Ahead-Of-Time (AOT) compilation, which improve runtime speed without sacrificing reliability.
- Fewer runtime checks: With static typing, the code is less likely to hit runtime type errors, which can slow or crash an application.
When Dynamic Typing Slows Things Down
Dynamic typing gives developers more flexibility, but that flexibility comes at a cost, especially in performance-heavy environments.
Here are common performance pitfalls seen in dynamically typed code:
- Runtime type checking adds overhead: Each time you assign or pass a variable, the interpreter has to verify its type, which uses CPU cycles.
- Harder for interpreters to optimize: Without fixed types, interpreters can't make assumptions. This limits how much they can optimize memory or execution flow.
- Garbage collection can become costly: Dynamically typed languages often rely on automatic memory management. When variables change types or structures frequently, memory gets harder to manage efficiently.
- Type confusion leads to slower functions: If a function receives inconsistent types, the interpreter may take longer to resolve how it should behave. Over time, this drags down performance.
Mitigating Speed Issues in Dynamic Languages
While dynamic languages aren't as fast out of the box, smart developers can avoid common traps. Here's how we improve performance in dynamic environments:
- Type hinting and static analysis tools: Using tools like MyPy for Python or TypeScript for JavaScript adds a layer of type safety and allows pre-runtime checks without losing flexibility.
- Optimized libraries and JIT runtimes: Python's PyPy and JavaScript's V8 engine use JIT compilation to speed up repetitive code by compiling parts of it to machine code on the fly.
- Code profiling and refactoring: Regularly running performance profiles helps identify slow functions or inefficient memory usage. We then refactor those areas, sometimes rewriting performance-critical parts in faster static languages.
Balancing Developer Speed and Code Safety
Choosing between static and dynamic typing isn't just a technical decision. It shapes how fast teams work, how they share code, and how easily new developers jump in.
The right balance depends on your team's goals, pace, and how much risk you're willing to take.
Dynamic Typing Advantages: Speed First, Questions Later
Dynamic typing gives developers freedom. You don't need to declare every variable's type or write long definitions before testing an idea.
That means:
- Faster coding: Developers can write less code to get things done.
- Easier prototyping: You can test features without getting blocked by type rules.
- Less boilerplate: There's no need to define every type, so the code stays clean and short.
For individual developers or small teams, that flexibility helps ideas move fast from whiteboard to working code.
Why Startups Prefer Dynamic Typing
Startups care about speed. They need to launch fast, test fast, and change direction quickly. Dynamic languages like Python, JavaScript, and Ruby make that possible.
Here's why many startups lean on dynamic typing:
- Time-to-market matters: Getting a working product out fast is more important than writing perfect code upfront.
- Short feedback loops: Developers can try ideas quickly and adjust based on user needs.
- Small teams, fewer blockers: In early stages, it's more efficient to move fast with fewer rules.
But while this freedom speeds up development, it can also lead to hidden bugs or technical debt if the team isn't careful.
Static Typing Benefits in Collaboration
Static typing shines in bigger teams or long-term projects. It enforces structure, which makes collaboration smoother.
Here's how:
- Clear contracts between code parts: Developers know what to expect from functions or variables.
- Faster onboarding: New team members can read the code and understand types right away.
- Better code reviews: Static typing helps reviewers spot problems early, without guessing what a function does.
- Fewer bugs in production: Type checks catch errors before the code runs.
When the team grows, this structure helps keep everyone on the same page.
When to Pick Static or Dynamic Typing for Your Project
Choosing between static and dynamic typing isn't just a technical decision. It depends on your project goals, team size, and how fast things need to move.
Let's break down when each approach makes the most sense.
Context Matters: One Size Doesn't Fit All
The best type system depends on your environment. Here's how we look at it:
- Startups need to be agile and quickly experiment with new ideas to stay competitive.
- Enterprises care more about stability, security, and long-term code maintenance.
- Project size plays a role. Small apps can afford to be flexible. Large systems need more control.
- Team skill level also matters. Junior developers may benefit from the guardrails of static typing.
Understanding these factors helps you make the right call.
When Static Typing Is the Smart Choice
If your project requires precision, static typing is your friend. It helps avoid bugs before the code runs and keeps things predictable.
It's the better choice when:
- The code will live for years: Static typing makes long-term maintenance easier.
- The domain is complex: Industries like fintech, healthcare, or aerospace demand strict checks.
- You have a large team: Clear type definitions make collaboration smoother.
- Reliability is critical: Fewer surprises mean fewer bugs in production.
When Dynamic Typing Makes More Sense
Sometimes, you need speed more than structure. That's where dynamic typing shines. It works best when:
- You're building an MVP: Time-to-market matters more than long-term code quality.
- The requirements are changing fast: Dynamic typing makes it easy to adjust.
- You have a small, experienced team: They can handle edge cases and move quickly.
- Prototyping is the priority: Prototyping is ideal for fast feedback and testing ideas.
Final Word
There's no "best" typing system for every project. Instead, there's a right choice for the moment. Dynamic typing helps you move fast. Static typing helps you stay safe.
Read Also: Common Mistakes When Building an MVP
Hybrid Typing: The Future of Flexible, Safe Code
In today's fast-paced software world, developers don't always want to choose between static and dynamic typing. That's why hybrid typing is gaining traction.
It blends the flexibility of dynamic typing with the safety of static checks, giving developers more control without slowing them down.
What Is Gradual Typing?
Gradual typing lets you add type hints to your code bit by bit. You start with dynamic typing and layer in type annotations as your project grows.
Tools like:
- TypeScript for JavaScript
- MyPy for Python
- Pyright for real-time Python checks
With gradual typing, you don't have to rewrite everything. You can type the most critical parts of your code first and leave the rest flexible.
Why Optional Typing Is on the Rise
Developers are increasingly embracing optional typing for greater flexibility and cleaner code. Developers appreciate how it supports both quick experimentation and long-term stability.
Languages like Python, JavaScript, and Ruby are seeing stronger tool support to make this easier.
What's driving the shift?
- Codebases are getting bigger
- Teams are more distributed
- Quality matters from day one
Modern IDE and Static Analysis Support
Today's development tools are built to help. With strong editor support, hybrid typing becomes practical and productive.
For example:
- Using TypeScript with VS Code makes development smoother with features like instant type checking, smart suggestions, and quick code fixes.
- IntelliJ IDEA and PyCharm show inline warnings and suggest type improvements.
- Pyright gives blazing-fast type checking for large Python projects.
These tools help boost confidence without requiring full conversion to a statically typed language.
Screenshots showing error highlights, in-editor type suggestions, and refactoring tips make the benefits clear to teams during onboarding or reviews.
Final Thoughts
Hybrid typing isn't just a trend. It's a smart approach to modern software development. You don't have to pick sides between speed and safety.
With tools like TypeScript, MyPy, and Pyright, you can get the best of both worlds.
Conclusion
Choosing between static and dynamic typing comes down to the needs of your project. If safety, long-term support, and clear collaboration are top priorities, static typing gives your team the structure it needs. But if speed, quick testing, and early flexibility matter more, especially for startups or prototypes, dynamic typing helps you move fast without getting bogged down.
There's no one-size-fits-all solution. The smart move is to match your typing strategy to your goals, timeline, and team skills. We recommend testing both in a limited scope. This gives you real data on what fits best with your workflow.
At CISIN, we've helped clients across industries, from healthcare to e-commerce, find the right balance. Our team knows when to push for type safety and when to stay flexible. We guide teams through these choices with real-world insight and technical experience.
Frequently Asked Questions (FAQs)
Can I mix static and dynamic typing in one project?
Yes, some modern languages like TypeScript (JavaScript with static typing) or Python with type hints allow you to blend both. This gives teams flexibility while gradually introducing safety checks.
Does static typing always make my code faster?
Not always. While static typing can enable optimizations, performance also depends on the language, compiler, runtime, and how the code is written.
Which typing strategy is better for AI or machine learning projects?
Dynamic typing is often preferred in early-stage AI/ML projects for rapid experimentation, but production-level models benefit from static typing for reliability and maintenance.
Is dynamic typing less secure than static typing?
Not inherently. Security depends more on how you write and test your code. However, static typing can help catch risky inputs or function misuse earlier.
What are the best tools for adding type safety to dynamically typed languages?
Some popular tools include TypeScript for JavaScript, MyPy for Python, and Sorbet for Ruby. These help catch type issues without changing the base language.
Do companies switch from dynamic to static typing as they scale?
Yes, it's common. Startups may begin with dynamic typing for speed but migrate to static systems as their codebase and team grow to ensure stability.
Which typing approach is easier for onboarding new developers?
Static typing often provides better documentation through code, making it easier for new developers to understand variable types and function expectations.
How do typing strategies affect testing needs?
Static typing reduces some types of bugs, which means fewer runtime tests for type-related issues. Dynamic typing usually requires more thorough testing to catch type mismatches.
Need Help Choosing the Right Typing Strategy?
At CISIN, we help teams find the best fit between static and dynamic typing based on their project goals. Whether you're building fast or scaling big, our experts are here to guide you.
Let's talk about what works best for your software.