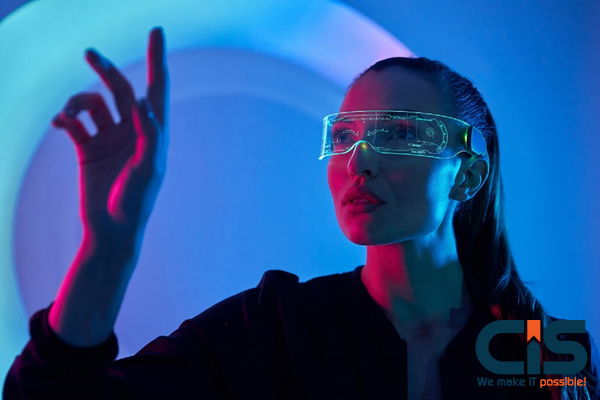
Functional programming is quickly becoming a go-to approach in modern software development. In 2025, a report found that 35% of enterprises are either using or actively exploring functional programming for real-world applications. This shift is happening because companies need code that's reliable, easy to scale, and less likely to break under pressure.
At its core, functional programming is a way of writing code using pure functions. These functions don't change things outside of themselves and always return the same result if you give them the same input. That means fewer surprises and easier debugging.
Unlike traditional coding styles that rely heavily on changing data and side effects, functional programming helps developers build cleaner, more dependable software. It also works really well for apps that need to handle a lot of users or process data quickly.
That's why more teams are choosing functional languages to build future-ready systems.
Understanding Functional Programming
Functional programming can sound complex at first, especially if you're used to writing code the traditional way. But the truth is, its core ideas are simple and easy to grasp when explained with real-world examples.
Let's break them down with simple examples so you can see and understand what are functional programming languages.
Read More: How Much Can Functional Programming Save You
Immutability
Immutability means once data is created, it doesn't change. Think of it like writing with a permanent marker. If you make a mistake, you don't erase, you start fresh. This helps avoid hidden bugs that come from unexpected data changes.
In functional programming, immutability makes your code safer and easier to understand. Every update creates a new version of the data, leaving the original untouched.
Pure Functions
Pure functions are like vending machines. Put in the same input, and you'll always get the same output, no matter what.
They don't rely on outside variables or change anything outside themselves. This makes them easy to test and reason about, especially in big systems.
First-Class Functions
In functional programming, functions are treated like values. This is called a first-class function.
You can store them in variables, pass them to other functions, or return them. It's like giving someone a to-do list, they can run it anytime. This makes your code more flexible and powerful.
Referential Transparency
Referential transparency means any function call can be replaced with its result, and the code still works the same.
Imagine replacing "2 + 2" with "4" in a math problem. It doesn't change the answer. In coding, this improves readability and makes bugs easier to spot.
Functional vs OOP and Procedural Programming
In object-oriented programming (OOP), objects often change over time. In procedural programming, shared data is common. Both can lead to bugs that are hard to trace.
Functional programming avoids this by using pure functions and immutable data. This leads to fewer surprises, more stable code, and better scalability.
Why These Concepts Matter
When building complex systems, these ideas help reduce bugs and improve code quality. You get cleaner code that's easier to test, maintain, and scale.
That's why more developers are choosing functional programming for modern software projects.
Deep Dive: Top Functional Programming Languages
It offers strong tools for building safe, scalable, and fast software. Below, we take a closer look at the most popular functional programming languages.
Each language has its strengths, practical uses, and helpful learning resources.
Whether you're a beginner or an experienced developer, this guide on functional programming languages will help you pick the right tool and start building with confidence.
Haskell
Haskell is a purely functional language. That means everything you write in Haskell follows strict functional rules. It uses lazy evaluation, which only runs code when it's needed.
This makes programs more efficient. Its strong static typing also catches many errors before you even run the code.
Where is it used?
Haskell is a favorite in areas like financial modeling, academic research, and even compiler development. It's ideal for systems that need to be both correct and stable.
How to learn it:
Start with the fun and easy-to-read book and work on small projects using Exercism or Codewars.
These will help you practice real problems.
Scala
Scala mixes the best of functional and object-oriented programming. It runs on the Java Virtual Machine (JVM), so you can use it anywhere Java works.
Scala also has type inference, which makes code shorter and easier to read.
Where is it used?
Scala powers big data systems like Apache Spark. It's also used to build backend services and concurrent applications that handle many users at once.
How to learn it:
Check out the official Scala documentation and start contributing to open-source Spark projects. You'll learn the language and its real-world uses at the same time.
Read Also: Big Data, The Ultimate Game Changer for Modern Industries
Elixir
Elixir is built on the Erlang VM, known for its rock-solid concurrency and fault-tolerance. Elixir makes it easier to build real-time apps that don't crash under pressure.
Where is it used?
You'll find Elixir behind many real-time chat systems, messaging apps, and high-traffic web platforms.
How to learn it:
Use the Phoenix framework to build simple web apps. Try creating a chat app that works in real time. It's a great way to learn Elixir's strengths.
Clojure
Clojure is a modern Lisp dialect that runs on the JVM. It uses immutable data structures by default, which keeps your code predictable and clean.
It also supports both functional and dynamic programming.
Where is it used?
Clojure works well for data analysis, artificial intelligence, and systems that need high concurrency.
How to learn it:
Explore REPL-driven development and solve fun challenges at 4Clojure. It's a hands-on way to build skills quickly.
F#
F# is a functional-first language that runs on the .NET platform. It features strong type inference and a clean, concise syntax, making it friendly for both beginners and enterprise teams.
Where is it used?
F# is used in financial software, data science tools, and business applications across Microsoft's ecosystem.
How to learn it:
Follow the guide F# for Fun and Profit and build simple tools like data analysis scripts or calculators to get started.
OCaml
OCaml blends functional and imperative programming, with a focus on performance and type safety.
It compiles to fast, native code and is used when speed matters.
Where is it used?
You'll find OCaml in fintech, compiler development, and even in formal verification systems where software must be mathematically proven to work.
How to learn it:
Read Real World OCaml and experiment by building small compilers or code analyzers to see its power.
Erlang
Erlang is built for systems that must run without failure. It supports soft real-time processing, fault tolerance, and lightweight process-based concurrency. That makes it perfect for reliable systems.
Where is it used?
It's popular in telecom, IoT systems, and instant messaging apps where uptime and speed are critical.
How to learn it:
If you're new to Erlang, begin by learning Elixir first. It's more beginner-friendly and built on top of Erlang. Then dive into Erlang's OTP framework to understand its core features.
This Comprehensive list of functional programming languages will help you understand this concept clearly.
Choosing the Right Functional Programming Language for Your Project
Picking the right functional programming language is key to your project's success. To make the best choice, start by looking closely at your project's specific needs.
Here are some important factors to consider:
Match Language to Project Requirements
Start by identifying the core technical demands of your project. This will help narrow down languages that offer the features you need most.
- Concurrency: If your project requires running many tasks at the same time, languages like Elixir and Erlang are built to handle concurrency well, ensuring smooth and efficient multitasking.
- Performance: For projects where speed and error prevention are crucial, Haskell and OCaml provide strong type safety and optimized performance, reducing bugs and improving reliability.
- Ecosystem and Platform Support: Some languages fit better depending on your current tech stack. Scala and Clojure work on the JVM, making them compatible with Java tools. F# integrates with Microsoft's .NET, ideal if you rely on that ecosystem.
Balance Learning Curve and Productivity
Every language comes with its learning demands. It's important to balance how quickly your team can learn a language with the productivity benefits it brings over time.
- Easier-to-learn languages let your team start coding quickly but may limit advanced features early on.
- More complex languages like Haskell might take longer to master but offer better long-term code quality and fewer bugs.
Learn from Real-World Projects
Practical experience shows that matching a language to the project's goals makes a real difference in results.
- One client used Scala to quickly build a scalable data pipeline, benefiting from Scala's integration with big data tools.
- Another client picked Elixir for its fault tolerance, successfully supporting a real-time chat app without downtime.
These prove that understanding project goals and constraints helps you pick the most effective functional language.
Effective Learning Strategies for Functional Programming
Learning functional programming can be a smooth and rewarding journey if you follow the right strategies.
Here are some practical tips to help you get started and keep progressing effectively.
Start with Hybrid Languages for a Smooth Transition
If you come from an object-oriented programming (OOP) background, diving straight into pure functional languages might feel overwhelming.
Hybrid languages like Scala and F# offer a gentle introduction because they mix functional and OOP styles.
This way, you can use familiar concepts while gradually learning functional programming principles.
Embrace Hands-On Learning
Functional programming concepts become clearer when you practice them directly. Use REPL (Read-Eval-Print Loop) environments to write and test code instantly.
Interactive tutorials also guide you through concepts step-by-step, making complex ideas easier to understand. Building small projects, like simple calculators or data processors, lets you apply what you've learned.
Engage with the Community
Learning doesn't happen in isolation. Join online forums related to functional programming, such as Reddit's functional programming groups or Stack Overflow.
Contributing to open-source projects on GitHub exposes you to real-world code and collaborative workflows.
Also, participate in coding challenges focused on functional programming to sharpen your problem-solving skills.
Use Trusted Books and Online Resources
Choosing the right study materials can make all the difference. Based on our training experience, authoritative books like Learn You a Haskell for Haskell beginners or Programming in Scala provide clear, reliable guidance.
Online platforms such as Exercism, Codecademy, and official documentation offer structured courses tailored to different skill levels.
Combining these resources with practice and community involvement creates a solid path toward mastering functional programming.
Debunking Common Myths About Functional Programming
Functional programming often gets misunderstood. Many think it's only useful in academic circles, too hard to learn, or slow in real-world use.
Many top tech companies use functional programming to build fast, reliable, and scalable systems.
Myth 1: "Functional Programming is Just for Academics"
This myth comes from the fact that functional programming has roots in math and theory. But today, companies like Twitter, WhatsApp, and Klarna use functional languages like Scala, Erlang, and Elixir in real products.
These tools help teams build complex systems that run reliably at scale.
Myth 2: "It's Too Hard to Learn"
Functional programming does feel different at first, especially if you're used to object-oriented languages. But many languages, like F# and Scala, offer a gentle learning curve by blending functional and traditional styles.
With hands-on projects and simple tools like REPLs, developers quickly pick up core concepts like pure functions and immutability.
Myth 3: "Functional Code Runs Too Slow"
This is no longer true. In fact, functional languages often outperform others in specific tasks. For example, Elixir (built on Erlang) powers real-time chat apps and messaging systems that handle millions of users.
Functional design encourages lightweight, stateless services that scale easily, often leading to better performance under load.
Real-World Success Stories Using Functional Programming
Functional programming isn't just for the classroom. Some of the world's most-used platforms rely on it every day.
These companies chose functional languages for one reason: they needed systems that were fast, reliable, and easy to scale.
Here are five success stories that prove functional programming works in the real world.
WhatsApp (Erlang)
Why Erlang?
WhatsApp needed a way to handle millions of messages without crashing. Erlang, designed for telecom systems, was a perfect fit. It supports lightweight processes and fault-tolerance by design.
The Result:
WhatsApp served over 2 million users per server in its early days with a very small engineering team. Erlang helped them keep uptime high and latency low, even under heavy loads.
Discord (Elixir)
Why Elixir?
Discord needed to deliver real-time communication to millions of gamers around the globe. Built on the Erlang VM, Elixir offered concurrency, fault tolerance, and scalability.
The Result:
Discord handles millions of simultaneous voice and chat connections every day. Elixir helped the platform grow fast while keeping infrastructure costs under control.
Bloomberg Terminal (Clojure)
Why Clojure?
Bloomberg needed a flexible, modern language for its terminal software. Clojure's immutable data structures and simple syntax made it a strong choice.
The Result:
Clojure powers part of the Bloomberg Terminal used by finance professionals worldwide. Its functional nature helps keep the system stable and easy to maintain over time.
Jane Street (OCaml)
Why OCaml?
Jane Street, a global trading firm, needed safety and performance in its financial software. OCaml's static typing and expressive syntax make it ideal for building large systems with minimal bugs.
The Result:
OCaml runs much of Jane Street's trading infrastructure. Its compile-time checks reduce runtime errors and help developers move faster with confidence.
Early Twitter Stack (Scala)
Why Scala?
When Twitter outgrew its original Ruby backend, it needed something faster and more scalable. Scala's blend of object-oriented and functional programming made the transition smoother.
The Result:
Twitter rebuilt key parts of its backend using Scala. This helped them reduce downtime and improve performance during periods of massive user growth.
Conclusion
So When the question arrives that what are functional programming languages, we learned that it is no longer a niche skill. It's a proven approach to building scalable, secure, and high-performance applications. From real-time messaging platforms to financial systems, many of today's most reliable software solutions are powered by functional languages. Companies that once struggled with scaling or reliability have seen measurable improvements after adopting functional programming.
The key is not just learning the theory but applying it. Start with small projects, explore hybrid languages like Scala or F#, and join communities that support growth and learning. By becoming fluent in both functional and object-oriented programming, developers build the flexibility needed to tackle a wider range of problems with the right tools.
The future of software development will favor teams that can adapt quickly and work with modern paradigms.
Frequently Asked Questions (FAQs)
Is functional programming better than object-oriented programming for web development?
It depends on the type of project. Functional programming languages are great for building applications that require high concurrency, reliability, or clean data flow. On the other hand, object-oriented approaches can be more intuitive for UI-heavy apps. In many real-world cases, a hybrid approach combining both paradigms is the most practical solution.
Can functional programming be used in mobile app development?
Yes, it can. While not the most common choice for mobile apps, functional programming languages like Elixir (with Phoenix LiveView) or functional-style Kotlin are used to build maintainable and scalable apps. They help manage state cleanly, especially in apps that rely on real-time data or complex logic.
What are the biggest challenges when adopting functional programming in a team?
Adopting functional programming languages often involves a mindset shift. Teams used to object-oriented or procedural code may find concepts like immutability and pure functions challenging at first. However, with mentorship, practical training, and small project pilots, teams usually adapt successfully.
Does functional programming work well with microservices architecture?
Yes. Microservices benefit from the stateless nature of functional programming languages. Since each service is isolated and uses pure functions, it's easier to test, debug, and scale. This makes FP a great match for distributed systems.
Are there job opportunities for functional programming developers?
Yes. While the job market for functional programming languages is more niche, there's strong demand in industries like finance, blockchain, healthcare, and data engineering. Roles that require knowledge of Haskell, Clojure, Scala, or Elixir often pay well due to the specialized skill set.
Is it harder to debug functional code compared to OOP code?
Not really. Functional programming languages promote pure functions and discourage side effects, which makes bugs easier to trace. Tools like REPLs and strong static type systems also catch errors early during development.
Can I use functional programming in mainstream languages like Java or Python?
Absolutely. Both Java (from Java 8 onwards) and Python support functional constructs like lambdas, map/filter/reduce, and immutability patterns. While they aren't purely functional, they allow developers to apply FP concepts effectively.
How does functional programming help in data-intensive applications?
Functional programming languages are well-suited for handling large volumes of data. They simplify data transformations using functions like map, reduce, and filter. The immutable nature of FP also ensures data consistency, which is vital for big data and parallel processing tasks.
Ready to boost your software with Functional Programming?
Whether you're new or experienced, our expert team at CISIN can help you build reliable and scalable solutions. Contact us today to discuss your functional programming needs and start your journey toward smarter software.