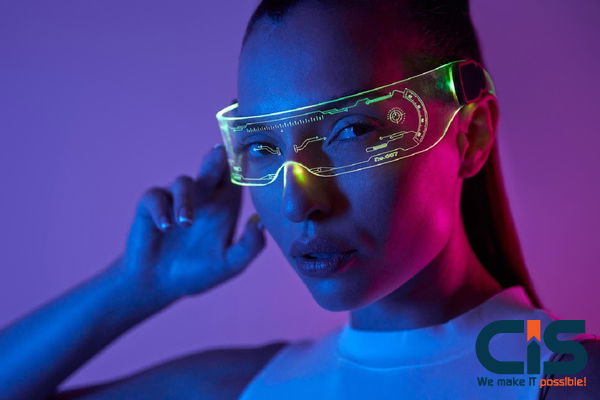
Python has earned itself the status of one of the world's favorite programming languages due to its flexibility and user-friendliness, making it popular with novice and advanced developers alike. If you are starting in programming or looking to hone your Python abilities further, this blog is for you. In particular, seven simple yet helpful tips will empower you to quickly write cleaner, more efficient Python code while saving time and enhancing your coding acumen.
These tips go to the core of Python programming, offering practical techniques and essential strategies that can make a significant difference to any Python project. They cover issues ranging from variable naming and list comprehensions, library utilization, and the art of keeping code DRY (Don't Repeat Yourself).
As part of our Python programming tutorial series, we will discuss the importance of adequately commenting on your code and taking advantage of Python's built-in functions. Lastly, unit tests should always be written to test code reliability. With these tips in your arsenal, you won't only write better Python code. Still, you will gain greater confidence as an expert programmer using this language.
Use Descriptive Variable Names
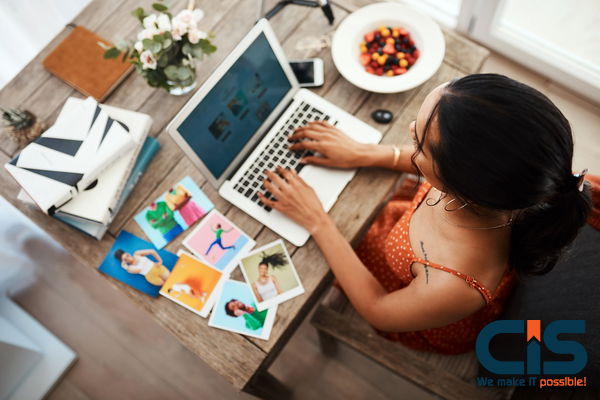
Python programming best practice recommends descriptive variable names as one of its fundamental tenets, rather than using single-letter or obscure variable names like "x" or "temp." Doing this makes your code readable while helping other developers better comprehend its intent.
Using descriptive variable names is essential in Python programming and all programming languages. It should always be practiced when selecting variable names to convey their purpose or data storage space clearly and succinctly. Not only is this beneficial to yourself as the programmer, but it's also invaluable when reading code from colleagues or collaborators - more on why this practice matters below.
Readability and maintainability: Incorporating descriptive variable names will make your code more accessible for others, including yourself, to read. Revisiting it helps people quickly grasp its contents without decoding cryptic or abbreviated words.
Self-documentation: Properly named variables serve as self-documenting comments within code, explaining what each variable represents or stores. By eliminating unnecessary inline comments and streamlining your code with this approach, your code becomes cleaner and more concise.
Error-prone code: Giving variables descriptive names lowers the risk of bugs due to misinterpreting or misconstruing their purpose; when you know exactly what each variable should do, making mistakes becomes far less likely.
Improved Collaboration: For programming teams working on collaborative projects, variable names that are clear and descriptive can enhance communication and teamwork among programmers by decreasing deciphering times for code written by each party and eliminating miscommunication between team members. By taking these steps, clear communication should lead to fewer misinterpretations of one another's code while eliminating potential misunderstandings between them.
Here's an example to illustrate the significance of descriptive variable names:
# Bad variable naming a = 10 b = 5 c = a + b # What does 'c' represent? # Improved variable naming initial_balance = 10 withdrawal_amount = 5 new_balance = initial_balance - withdrawal_amount # Clearly conveys the purpose
In the second example, descriptive variable names show that the code performs a simple financial transaction. This clarity simplifies the understanding of the code, and you can easily tell that it calculates the new account balance after a withdrawal.
Using descriptive variable names in your Python code is a small but highly impactful practice. It enhances code readability, reduces errors, and improves collaboration, leading to more maintainable and efficient software development. Therefore, it's a practice that every Python programmer, from beginners to experts, should embrace in their coding journey.
Embrace List Comprehensions
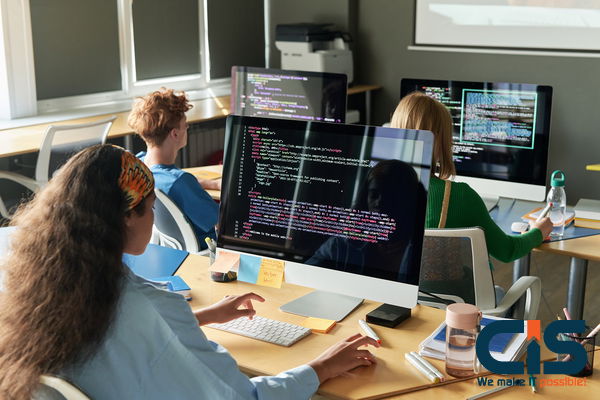
Python list comprehensions offer an elegant solution for quickly creating lists. They let you operate on each element in an iterable, creating new tables with just one line of code. Utilizing list comprehensions in your programming makes code efficient and readable, helping improve productivity.
Introduction to Python programming requires mastery of list comprehensions - they offer a simple yet powerful means for creating lists by applying an expression to each item of an iterable, such as a list, tuple, or range. Here are several key reasons to adopt list comprehensions as an integral component in your Python code:
Conciseness: List comprehensions enable you to write concise and expressive code by eliminating verbose loops and providing similar functionality through one line of code - significantly increasing readability and maintainability.
Readability: List comprehensions make your code more readable by offering clear syntax that even experienced Python developers find easy to comprehend, thus enabling beginners in Python to grasp its purpose quickly.
Efficiency: List comprehensions are both elegant and efficient; their implementation in Python has been optimized to maximize speed, making them faster and more economical in many instances than traditional loops.
Functional programming: List comprehensions align with the principles of available programming by emphasizing immutability and representing operations on data as transformations; this approach can lead to cleaner code with reduced instances of error.
Consider this simple example where we want to build a list of squared numbers from 1 through 5 using list comprehensions:
Using a for loop:
squared_numbers = [] for num in range(1, 6): squared_numbers.append(num ** 2)
Using a list comprehension:
squared_numbers = [num ** 2 for num in range(1, 6)]
The list comprehension version is more concise, readable, and efficient. It conveys the intention clearly: to generate a list of squared numbers from a given range.
Embracing list comprehensions is a valuable skill for Python programmers. They can help you write cleaner, more concise, and readable code while improving code efficiency. By mastering this feature, you can make your Python code more elegant and aligned with the language's philosophy of readability and simplicity.
Leverage Python Libraries
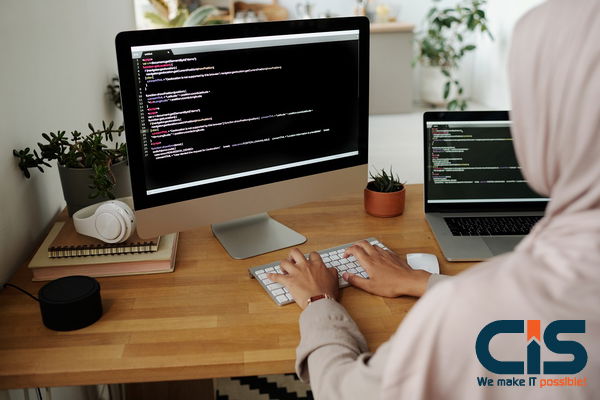
Python offers an expansive ecosystem of libraries and packages to save you time and effort when working with data, performing web scraping operations, creating graphic user interfaces, or performing machine learning tasks. Famous examples are NumPy, pandas, Tkinter, and scikit-learn.
Leveraging Python libraries is critical to becoming a more proficient and efficient programmer. Python offers an abundance of libraries and packages that extend its capabilities across numerous fields - data analysis, web development, and machine learning - making leveraging these libraries essential. Here's why this strategy matters so much:
Reusability: Python libraries provide pre-written code modules designed to address common programming tasks and challenges, making use of them an efficient way of tapping into the expertise and work of the wider Python community. Doing this saves time and effort as it eliminates coding everything yourself from scratch.
Efficiency: Python libraries are highly optimized and well-tested, providing efficient implementations of complex operations for more responsive code that reduces resource usage. Their efficiency becomes especially essential in large-scale or resource-intensive apps where efficiency becomes critical in meeting user demand.
Specialized functions: Many Python libraries provide unique functions and tools tailored for specific tasks, like numerical operations using NumPy or data manipulation using pandas libraries. Utilizing such specialized features enables programmers to write more robust and sophisticated apps.
Quality and security: Established Python libraries are regularly upgraded for performance, security, and bug fixes - meaning your code can take advantage of these ongoing improvements in quality and safety. Leveraging established libraries ensures your code can fully utilize these constant upgrades to keep pace with modernization efforts.
Learning opportunities: When using Python libraries, problems are solved, but you also gain knowledge from their documentation and source code that provides best practices and industry-standard approaches to incorporate into your programming journey. This educational asset could be beneficial as your journey advances with Python programming.
Imagine creating a web app. Instead of starting from scratch, Flask or Django libraries provide pre-packaged components for managing HTTP requests, routing data through servers, and rendering templates quickly, allowing developers to focus their development time and attention on building out specific aspects of their app.
Leveraging Python libraries is a core practice of Python programmers. By understanding which libraries exist and how best to employ them, programmers can take full advantage of Python and create more efficient, feature-packed apps with more significant potential than ever.
Keep Code Dry (Don't Repeat Yourself)
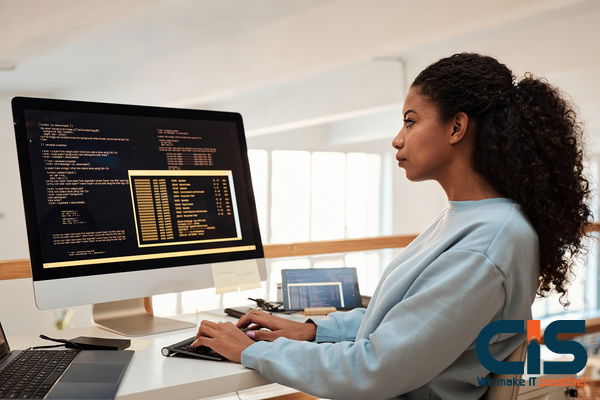
The DRY principle is an essential concept in programming that emphasizes reducing redundancy in code. Whenever you duplicate code across several places, consider creating functions or classes to encapsulate that logic and reduce duplication while making your code more maintainable.
"DRY," short for "don't repeat yourself", is a fundamental principle in software development - particularly Python programming - encouraging programmers not to duplicate code while keeping their codebase as concise and manageable as possible. Being mindful about keeping DRY is incredibly essential. Here is why keeping your code DRY matters:
Reduce code duplication: Duplication is one of the primary sources of errors and maintenance challenges, making it harder to ensure consistency across platforms and fix bugs quickly and reliably. By keeping your code DRY, redundancies are eliminated for more excellent reliability of software systems.
Ease of maintenance: DRY code can be easier to manage since changes or updates only need to be applied once, making debugging and updating simpler and decreasing risks related to duplicate code updates.
Improved readability: DRY code tends to be easier for readers to follow and interpret, especially in complex projects with multiple pieces of repetitive code that are hard to comprehend, by consolidating them all together into one central place, making understanding much clearer.
Consistency: Reusing code through functions, classes, or modules ensures consistency in its behavior and appearance across your codebase. Having one authoritative source for every functionality across all your modules and styles makes it much simpler to reach predictable and standardized results across your code.
As an illustration of DRY code writing, consider an everyday example. You need to calculate the area of a rectangle multiple times throughout your code - instead of typing out that formula each time manually, why not define a function instead?
def calculate_rectangle_area(width, height): return width * height
With this function, you can calculate the area of a rectangle by calling calculate_rectangle_area(width, height) wherever you need it in your code. This eliminates code duplication and makes the code more readable and maintainable.
Adhering to the DRY principle is a best practice that leads to more efficient, maintainable, and less error-prone code. Python programmers can create more accessible software to develop, understand, and extend by reusing code through functions, classes, and modules and avoiding repetition.
Properly Comment Your Code
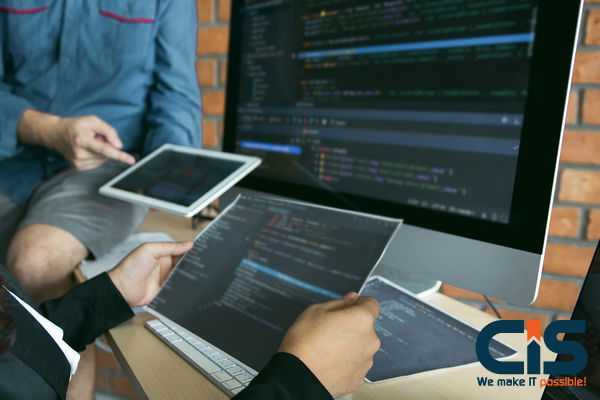
Adequate documentation is crucial in Python programming. Adding comments to your code can help you and others understand its purpose and functionality more quickly. Python supports single-line comments (using #) and multiline words using triple quotes. However, over commenting should be avoided; nonetheless, clear and concise commentary may prove indispensable.
Commenting your code correctly is an integral aspect of Python programming, as it helps developers better comprehend its purpose, functionality, and design decisions. Here's why adding Python program comments is vital:
Documentation: Your comments serve as documentation of your code. They provide insight into what it does, its function, and any decisions that make understanding and maintaining it more accessible in the future. Clear documentation can make you and others needing to read or keep your code much more straightforward.
Clarity and readability: Appropriate comments can dramatically enhance code clarity and readability. By offering context and explanation for code sections, they make it much simpler for you and others to comprehend the logic and intentions behind its creation.
Debugging and troubleshooting: Comments are an invaluable way of debugging when encountering errors or bugs in code, allowing you to follow the flow of your program more quickly, thus quickly pinpointing where any potential issues lie.
Communication: Writing code can often be an iterative effort; comments help team members communicate more efficiently by explaining the purpose and functionality of code components and also serve as an aid in conveying your thought processes to others when reviewing or explaining your code.
Maintainability: Over time, your code may need updating or modification; proper comments guide how to do so without breaking existing functionality - something which becomes especially helpful if revisiting an old project months or years after first writing it.
Here is an example to demonstrate the significance of appropriate commenting:
# Bad Commenting result = 0 # Initialize result to 0 for i in range(1, 6): result += i # Add 'i' to 'result' # Improved Commenting # Calculate the sum of numbers from 1 to 5 result = 0 for i in range(1, 6): result += i
In the second example, comments provide context and clarity about the purpose of the code, making it easier to understand.
Proper code commenting is an essential aspect of Python programming. It enhances code readability and aids in debugging and troubleshooting. It ensures your code is maintainable and understandable to you and your fellow developers. While it's crucial to balance commenting enough to be helpful and over-commenting, effective use of comments can be a powerful tool for creating high-quality Python programs.
Learn and Use Python's Built-in Functions
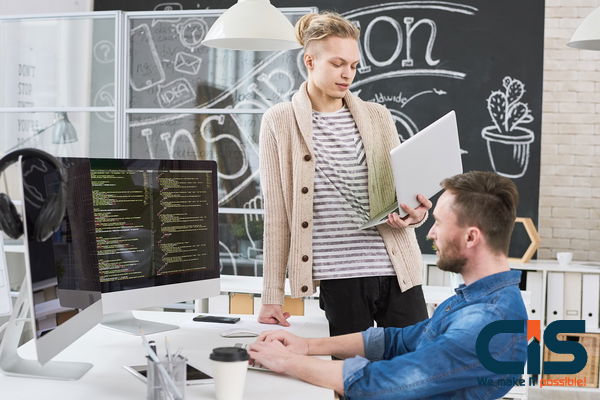
Python provides an impressive collection of built-in functions designed to simplify everyday programming tasks, including len(), max(), and min(), as well as sorted(), join(), and enumerate() functions. Understanding and employing these can make your code more efficient while eliminating the need to reinvent the wheel.
Learning Python's built-in functions is critical to becoming proficient with its programming. There is an impressive assortment of built-in functions explicitly designed to simplify everyday programming tasks; therefore, you must become acquainted with them and implement them into your code. Here's why learning and using them correctly are so essential:
Efficiency: Python's built-in functions are carefully optimized for performance, making use of them rather than writing custom solutions a more cost-efficient option than writing custom solutions yourself. Their finely tuned nature over time has led them to become reliable and quick solutions for many joint operations.
Code readability: At the core of Python are built-in functions that help make code more readable for you and other programmers working on projects together. These built-in functions make your code much more straightforward to read and comprehend - especially crucial when working collaboratively on collaborative endeavors.
Expressiveness: Python's built-in functions are expressive and can be used elegantly yet concisely to express complex operations, leading to cleaner and easier maintainable code. This conciseness can lead to cleaner code.
Standardization: By adhering to Python's standard library conventions and using preloaded functions, your code remains consistent and adheres to best practices widely accepted among its community of Python enthusiasts.
Below are examples to demonstrate their usefulness: len(), max(), and min() are some common built-in functions used in programming languages that can help manage text data efficiently. Here's some information regarding their function usage :
Example 1: Using len() to Get the Length of a List
names = ["Alice," "Bob", "Charlie"] length = len(names) print("Number of names:", length)
Related:- What Is the Process of Custom Software Development Consulting?
Example 2: Using sorted() to Sort a List
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5] sorted_numbers = sorted(numbers) print("Sorted numbers:", sorted_numbers)
In both examples, the built-in functions (len() and sorted()) simplify the code and make it more readable while ensuring correctness and efficiency.
Learning and using Python's built-in functions is essential for Python programmers. These functions save time, enhance code readability, and promote code consistency. By mastering these functions and knowing when and how to use them, you can write more efficient and elegant Python code.
Write Unit Tests
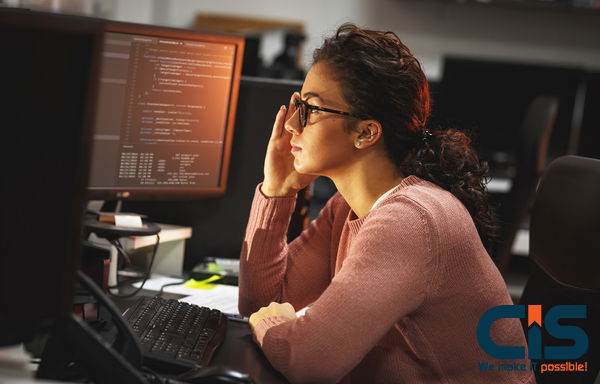
Unit testing is an integral components of software development. Python includes its unittest framework as a built-in testing library; other popular testing libraries like pytest provide another method. Writing unit tests ensures your code functions according to expectations while helping detect bugs early and quickly fix any potential problems, making your code more reliable and maintainable.
Writing unit tests is generally integral to Python programming and software development. Unit testing entails creating small tests designed to check individual units (elements) of code to make sure it functions as expected - hence why writing them is vital:
Bug detection: Unit tests provide an early means for spotting errors early in development processes. By validating that each unit of code performs as desired, unit tests help detect issues before they spread across multiple layers.
Refactoring assistance: Unit tests offer crucial support when making changes or refactoring existing code. When you modify any unit, its associated unit tests help ensure that any modifications don't create new issues elsewhere within your codebase.
Documentation: Unit tests serve as documentation of your code. By clearly outlining the expected behavior of components and their roles within an overall program, unit tests provide documentation for both you and other developers, assisting in understanding its purpose and requirements.
Quality assurance: Unit tests are essential in assuring code quality and maintainability. By creating unit tests for individual units, you're instilling good programming practices while ensuring they satisfy requirements set by you and others.
Regression testing: When code changes occur, unit tests should automatically rerun to ensure previously working components continue working without regressions. This guarantees continuity.
Python offers its unittest library as an effective means for writing unit tests; other testing frameworks like pytest may also be utilized; when writing unit tests in this language, test cases that verify individual units, such as functions or methods, should be created to test the functionality of these code blocks.
Here's an easy example of writing unit tests using the unittest library:
import unittest # Function to be tested def add(a, b): return a + b # Test case class TestAddFunction(unittest.TestCase): def test_add_positive_numbers(self): result = add(3, 5) self.assertEqual(result, 8) if __name__ == "__main__": unittest.main()
In this example, we create a test case for the add function, verifying that it correctly adds two positive numbers. Running this test will help ensure that the add function works as intended.
Writing unit tests is a fundamental practice for Python programmers. It improves code quality, helps detect and prevent bugs, and supports code maintenance and refactoring. You can build more reliable and robust Python applications by incorporating unit tests into your development process.
Conclusion
Python is a highly flexible programming language. By following these seven straightforward yet helpful guidelines, you can improve your Python programming abilities and become a more efficient and effective programmer. As part of your journey through Python development, remember that practice, experimentation, and reflection on past errors will ultimately bring success in building excellent code.
These seven simple yet crucial Python programming tips will undoubtedly enhance both your abilities and code quality, so let's quickly recap their key takeaways:
Use descriptive variable names: Selecting meaningful variable names will make your code more readable, understandable, and less error-prone - an essential practice in maintaining clean and maintainable Python code.
Accept list comprehensions: List comprehensions are an efficient and cost-effective method to generate lists, offering improved code readability while making complex operations less cumbersome.
Leverage Python libraries: Python offers an expansive library ecosystem that can save time and effort by providing specific functions for certain tasks, helping increase productivity while expanding project capabilities. Take Advantage Of Python Libraries
Code your code (Dry Your Code): Prevent code duplication by enclosing repetitive logic within functions or classes to minimize duplicating sense, thus decreasing errors while improving code reusability and maintainability. This strategy not only reduces error risk but also promotes code reusability.
Properly comment your code: Commenting your code provides valuable documentation, enhances code clarity, and assists debugging efforts. Clear and precise comments are crucial when working collaboratively on code projects.
Learn and utilize python's built-in functions: Python offers many built-in functions designed to increase code efficiency, readability, and consistency - familiarizing yourself with these functions is the cornerstone of effective Python programming. Familiarizing yourself with them properly and knowing when and how to apply them effectively are vital skills for effective programming using this language.
Develop unit tests: Writing unit tests will ensure that individual components of your code function as expected and help identify bugs early, support code refactoring efforts, provide documentation, and ultimately result in increased quality and reliability of code.
Want To Know More About Our Services? Talk To Our Consultants!
As part of your Python programming practices, you will create more efficient, maintainable, and dependable code by adopting these tips. Doing so will strengthen your coding abilities and make you a more experienced Python developer capable of taking on various programming challenges head-on.