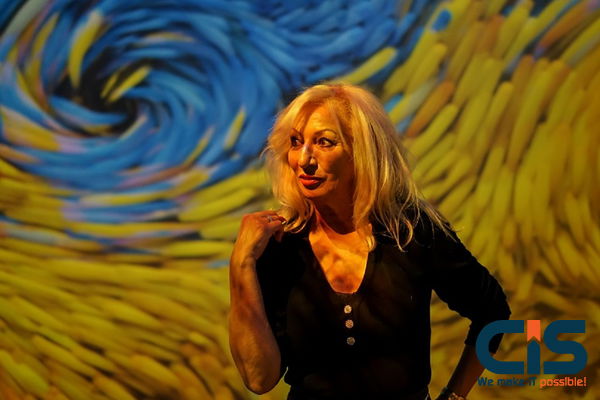
Globally, the software market is anticipated to increase at an impressive rate. By 2025, it is projected that this market segment would bring in an incredible $742.58 billion. With so many people learning to code, understanding the basics programming languages are more important than ever.
So, what is a programming language? Simply put, it's a way to give instructions to a computer. Just like we use English or Spanish to talk to each other, developers use code to tell computers what to do.
If you're new to coding, knowing the different programming languages helps you pick the right one for your goals. Some are great for web apps, others for data, games, or even robots.
Programming languages are how programmers may interact with computers and give them instructions on how to accomplish particular tasks.
They are used by developers to create websites, apps, and software, much like people use languages like English or Spanish to communicate.
This beginner's guide will walk you through the main programming language types with simple examples. Whether you're just curious or planning to start a tech career, this post will help you get started the smart way.
What Are Programming Languages?
A programming language is how people talk to computers. It's a set of rules and commands used to tell a computer what to do. Instead of using spoken words, we write code using special words and symbols.
In software development, programming languages help build everything from websites and mobile apps to games and smart devices. Each line of code gives the computer a task, like showing a button or calculating numbers.
Think of it like this: natural languages, such as English or Spanish, help people talk to each other. Programming languages do the same thing, but with machines. Instead of saying, "Turn on the light," you write a command the computer understands.
There are many programming languages, and each one has its strengths. As a beginner, learning what are the programming languages can help you choose the right path, whether it's for web design, data science, or mobile app development.
Compiled vs. Interpreted Languages
Another way to categorize programming languages is by how they're executed: compiled or interpreted.
Compiled Languages: Built Before Running
Compiled languages are translated into machine code before they run. You write the code, then a compiler converts it into a file that the computer can execute directly. This makes the program run faster but requires a compilation step.
C++ is a compiled language used for high-performance tasks like video games or simulations. Java is also compiled, but it runs on a virtual machine, making it versatile for apps across different devices.
Why it matters: Compiled languages are ideal for projects where speed and efficiency are critical. They're common in software development and large-scale systems, but can feel complex for beginners due to the compilation process.
Interpreted Languages: Running Line-by-Line
Interpreted languages don't need a separate compilation step. Instead, an interpreter runs the code line by line as you write it. This makes it easier to test and debug, though it can be slower than compiled languages.
Python is a popular interpreted language, great for quick projects like data analysis or automation scripts. Ruby, used for web development, is another example, known for its simplicity and readability.
Why it matters: Interpreted languages are beginner-friendly because you can see results instantly without extra steps. They're perfect for learning to code or building prototypes quickly.
General-Purpose vs. Domain-Specific Languages
Programming languages can be split by their scope: general-purpose or domain-specific.
General-Purpose Languages: Do It All
General-purpose languages are versatile and can be used for a wide range of projects. They're like a Swiss Army knife for coding-flexible and adaptable.
Python is a general-purpose language used for everything from web apps to machine learning. Java is another popular language for enterprise software, Android apps, and even web servers. These languages give you the freedom to explore different types of programming.
Why it matters: General-purpose languages are great for beginners because they let you try various projects without learning a new language each time. They're also in high demand in the job market.
Domain-Specific Languages: Built for One Job
Domain-specific languages (DSLs) are designed for specific tasks. They're less flexible but highly efficient for their intended purpose.
SQL is a DSL for managing database, you write queries to store or retrieve data, like customer records. HTML is another tool used to structure content on websites, like headings and paragraphs. These languages focus on doing one thing well.
Why it matters: DSLs are useful when you need to solve a specific problem, like building a website or analyzing data. They're often simpler for their niche but limited outside it.
High-Level vs. Low-Level Languages
Programming languages fall into two broad groups based on how close they are to human or machine language: high-level and low-level.
High-Level Languages: Easy for Humans
High-level languages are designed to be easy to read and write, even if you're new to coding. They use words and syntax that feel closer to everyday English, so you don't need to know how computers process instructions. For example, in Python, you might write print("Hello, World!") to display text. It's straightforward and beginner-friendly.
JavaScript is another high-level language, perfect for adding interactivity to websites, like making buttons respond to clicks. These languages handle a lot of the technical details for you, so you can focus on building your program.
Why it matters: High-level languages are great for beginners because they're easier to learn and use. They're widely used for web development, data analysis, and apps, making them a smart starting point.
Low-Level Languages: Closer to the Machine
Machine code, the native language of computers, is more closely related to low-level languages. They give you more control over how the computer uses its resources, like memory or processing power. However, they're harder to read and write because they use less human-friendly syntax.
Assembly is a low-level language where you write instructions that directly control the computer's hardware. C is another example-it's low-level but slightly easier than Assembly, used for tasks like building operating systems or embedded devices.
Why it matters: Low-level languages are powerful for tasks that need speed or direct hardware control, like game engines or system software. They're less beginner-friendly but show how computers work under the hood.
Programming Language Paradigms: A Beginner's Guide
When you start learning to code, you'll hear about different types of programming languages. But what makes them unique? It's not just their syntax or popularity, it's the way they approach solving problems.
These approaches, called programming paradigms, shape how a language works and what it's best used for.
In this section, we'll explore the main programming paradigms, explain how they influence language design, and give you clear examples to make it all click.
What Are Programming Paradigms?
A programming paradigm is like a blueprint for writing code. It's the method or style a language uses to solve problems.
Each paradigm has its own rules and logic, which affect how you write programs and what the language excels at.
Understanding these paradigms helps you pick the right language for your goals. Let's dive into programming languages and their paradigms.
Procedural Programming: Step-by-Step Problem Solving
Procedural programming is all about following a clear sequence of steps. You write instructions that the computer executes one after another, like a recipe. This paradigm is concerned with processes (or functions) that carry out particular tasks. It's straightforward and great for tasks that need a logical, step-by-step approach.
Languages like C and Fortran use this paradigm. For example, in C, you might write a program to calculate a sum by defining a function that adds numbers in a specific order. It's easy to follow and works well for tasks like system programming or simple calculations.
Why it matters: Procedural programming is intuitive for beginners because it mimics how we solve problems in everyday life. It's a foundation for many other paradigms and is still widely used in industries like engineering and software development.
Object-Oriented Programming: Organizing Code with Objects
Object-oriented programming (OOP) takes a different approach. It organizes code around "objects" rather than just functions. An object is like a mini-program that combines data (like variables) and actions (like functions) into one unit. Think of it as a car: it has parts (like wheels or an engine) and behaviors (like driving or braking).
Languages like Java and C++ are built for OOP. In Java, you might create a "Car" object with properties like color and speed, plus methods to accelerate or stop. This makes it easier to manage complex programs, like apps or games, where many parts interact.
Why it matters: OOP is great for building large, reusable, and scalable programs. It's widely used in software development, from web apps to mobile games, because it keeps code organized and easier to maintain.
Functional Programming: Focus on Functions and Immutability
Functional programming is a bit different. It treats coding like math, focusing on pure functions-small, predictable pieces of code that always give the same output for the same input. It avoids changing data (called immutability) and emphasizes solving problems with functions that don't rely on external variables.
Languages like Haskell and Scala shine here. For instance, in Haskell, you might write a function to double a list of numbers without altering the original list. This makes code more predictable and easier to test, especially for tasks like data analysis or machine learning.
Why it matters: Functional programming is gaining popularity because it reduces bugs and works well with modern technologies like artificial intelligence. It's a bit trickier for beginners, but it teaches you to think differently about coding.
Scripting Languages: Quick and Flexible Automation
Scripting languages are designed for speed and flexibility. They let you write short scripts to automate tasks or add functionality to apps. Unlike other paradigms, scripting languages are often interpreted, meaning they run line-by-line without needing to be compiled first. This makes them fast to write and test.
JavaScript and PHP are prime examples. JavaScript, for instance, powers interactive features on websites, like buttons or forms. You can write a quick script to validate a user's input on a webpage without rebuilding the entire site. PHP is often used for server-side tasks, like handling web forms.
Why it matters: Scripting languages are beginner-friendly because they're quick to learn and see results. They're perfect for web development or automating repetitive tasks, like renaming files or scraping data.
Logic Programming: Solving Problems with Logic
Logic programming is less common but powerful for specific tasks. It uses formal logic to define rules and let the computer figure out the solution. Instead of writing step-by-step instructions, you describe the problem's rules, and the language solves it for you.
Prolog is a classic example. In Prolog, you might define rules about family relationships (like "if X is a parent of Y, then X is a mother or father"). The program then answers questions based on those rules, like "Who is John's mother?" This is ideal for applications like artificial intelligence or expert systems.
Why it matters: Logic programming is niche but valuable for fields like AI, natural language processing, and database querying.
How Paradigms Shape Language Design
Each paradigm influences how a language is built and what it's used for. For example, C's procedural design makes it fast and efficient for system programming, while Java's OOP structure suits large-scale applications like banking software. Functional languages like Haskell are designed for reliability, making them great for data-heavy tasks. Scripting languages prioritize ease and speed, while logic-based languages like Prolog focus on reasoning.
When choosing a language, think about what you want to build. Are you creating a website? JavaScript's scripting paradigm might be your best bet. Working on AI? Functional or logic programming could be ideal. Understanding what are the different types of programming languages and their paradigms helps you make smart choices.
Why Paradigms Matter for Beginners
As a beginner, you don't need to master every paradigm right away. Start with one that matches your goals. Procedural and scripting languages like Python or JavaScript are often easier to pick up. As you grow, exploring other paradigms, like OOP or functional programming, will make you a more versatile coder.
By understanding these paradigms, you'll see why there are so many types of programming languages and how they fit into the coding world. It's like learning the rules of different sports; each has its style, but they all help you play the game better.
Some Popular Programming Languages
When you're starting to code, choosing the right programming language can feel like picking a favorite song from a massive playlist.
Each of the popular programming languages serves a purpose in the tech world. Python and JavaScript are fantastic starting points due to their simplicity and versatility. Java, C, and C++ are great for diving deeper into software or game development. SQL and R open doors to data-related careers, while languages like Swift or Go offer niche opportunities.
From building websites to analyzing data, these languages power the tech world. Let's explore what are the different types of programming languages and how they can kickstart your coding journey.
Python: The Beginner's Best Friend
Python is like the Swiss Army knife of programming: versatile, easy to learn, and widely used. Its simple, English-like syntax makes it a top choice for beginners. You can write a program to print "Hello, World!" in just one line: print("Hello, World!").
What it's used for: Python shines in web development (think websites like Dropbox), data science (analyzing trends or building AI models), and automation (like scripting tasks to rename files). For example, a beginner might use Python to create a simple web app with frameworks like Flask or automate data entry tasks.
Why it matters: Python's readability and huge community make it perfect for new coders. Its versatility means you can explore many fields without switching languages.
JavaScript: Bringing Websites to Life
JavaScript is the preferred programming language for creating dynamic websites. If you've ever clicked a button on a webpage and seen a pop-up or animation, JavaScript was likely behind it. It runs in your browser, so you don't need extra software to start coding.
What it's used for: JavaScript powers dynamic websites, like adding real-time chat to a site or creating interactive forms. For example, you could write a script to show a "Thank You" message when a user submits a form. It's also used in server-side development with tools like Node.js.
Why it matters: If you want to build websites or web apps, JavaScript is essential. Its immediate feedback (like seeing changes in a browser) makes it exciting for beginners.
Java: The Enterprise Workhorse
As Java is a stable, platform-independent language, any device that has a Java Virtual Machine (JVM) may run your code.
Its syntax is a bit more complex than Python's, but it's a powerhouse for big projects.
What it's used for: Java is popular for enterprise applications (like banking software) and Android app development. For instance, you could build an Android game or a backend system for a company's website using Java. It's also used in large-scale systems like Apache Hadoop.
Why it matters: Java's reliability and widespread use make it a valuable skill. It's a great choice if you're interested in mobile apps or corporate software, though it has a steeper learning curve.
Also Read: Why Java is the No. 1 Choice?
C/C++: Speed and Power
C and C++ are high-performance languages that give you control over a computer's hardware. They're not as beginner-friendly as Python, but they're critical for tasks that demand speed. C++ builds on C by adding object-oriented features.
What it's used for: These languages are used for system programming (like operating systems) and game development (think Unreal Engine games). For example, you could write a program in C++ to manage memory for a video game or build drivers for hardware.
Why it matters: C and C++ teach you how computers work at a low level. They're ideal for performance-critical projects and are great for coders who want to dive into technical challenges.
SQL: Talking to Databases
SQL (Structured Query Language) is a domain-specific language for managing databases. It's not for building apps but for storing, retrieving, and organizing data. If you've ever searched an online store's inventory, SQL likely powered that query.
What it's used for: SQL is used to manage databases, like storing customer data or retrieving product details. For example, you could write a query like SELECT * FROM products WHERE price < 50 to find all items under $50. It's essential for data-driven apps and business systems.
Why it matters: SQL is simple to learn and critical for data-related careers. Beginners can quickly grasp its basics and see real-world applications in web or business projects.
R: Crunching Numbers and Visuals
R is a language built for statistics and data visualization. It's less general-purpose than Python but excels in specific fields. Its syntax is straightforward for data tasks, making it approachable for beginners interested in analytics.
What it's used for: R is used for statistical analysis and creating charts or graphs. For example, you could use R to analyze survey data and create a bar chart showing results. It's popular in academia, finance, and data science.
Why it matters: R is a great choice if you love numbers or want to work in data-heavy fields. Its focus on visualization helps beginners create impressive outputs with minimal code.
Other Notable Languages
Several other languages are worth mentioning, each with unique strengths:
- Ruby: Known for its simplicity, used in web development (e.g., with Ruby on Rails for sites like Airbnb).
- PHP: Powers many websites (like WordPress), great for server-side web tasks.
- Swift: Apple's language for iOS apps, used to build apps like those on your iPhone.
- Kotlin: A modern language for Android development, often preferred over Java for its simplicity.
- Go: A fast, lightweight language from Google, used for cloud systems and scalable apps.
Also Read: 5 Tips For Mastering PHP
Recommended Languages for Specific Goals
Different projects call for different types of programming languages. Here are some popular goals and the best languages to start with for each.
Web Development: JavaScript, HTML/CSS, Python
If you want to build websites, these languages are your go-to:
- JavaScript: Essential for making websites interactive, like adding buttons or animations. For example, you could create a form that validates user input in real-time.
- HTML/CSS: Not programming languages in the traditional sense, but critical for web development. HTML structures content (like headings), while CSS styles it (like colors and layouts). You'll use these alongside JavaScript.
- Python: With frameworks like Django or Flask, Python is great for building the backend of websites, such as handling user logins or processing data.
Data Science: Python, R
If you're into analyzing data or building AI models, these languages shine:
- Python: Its libraries, like Pandas and TensorFlow, make it perfect for data analysis, machine learning, and visualization. For example, you could analyze sales data to predict trends.
- R: Designed for statistics, R excels at creating charts and running complex analyses. It's great for beginners who want to visualize data, like plotting survey results.
Mobile Apps: Swift (iOS), Kotlin (Android)
If you want to build mobile apps, focus on these:
- Swift: Apple's language for iOS apps, used for iPhone and iPad applications. You could build a simple to-do list app with Swift in a few hours.
- Kotlin: Google's preferred language for Android apps, known for its modern, concise syntax. It's great for creating apps like games or fitness trackers.
Game Development: C++, C#
If gaming is your passion, these languages are key:
- C++: A high-performance language used in game engines like Unreal Engine. You could write code to control a character's movement in a 3D game.
- C#: Popular with Unity, a beginner-friendly game engine. C# is great for building 2D or 3D games, like a platformer or puzzle game.
Also Read: 8 Programming Languages Dominating Popular Websites
Conclusion
Learning about the different programming languages can feel like stepping into a vast, exciting world.
From high-level languages like Python to low-level ones like C, and from procedural to object-oriented paradigms, each language and approach offers unique ways to solve problems and build amazing things.
In this guide, we've explored what are the different types of programming languages, how their paradigms shape their design, and which ones suit popular goals like web development, data science, mobile apps, or game development. Now, it's time to take the next step.
The key takeaway? You don't need to master every language or paradigm right away. Start with one that matches your interests, Python for its simplicity, JavaScript for web projects, or another that sparks your curiosity.
Each language opens doors to new skills and opportunities. The tech world is full of possibilities, and the best way to learn is by experimenting. Write a small program, build a simple project, or join a coding community to keep growing.
Frequently Asked Questions (FAQs)
Can I learn multiple programming languages at once?
You can, but it's not the best idea for beginners. Learning one language, like Python or JavaScript, helps you grasp core coding concepts without feeling overwhelmed. Once you're comfortable, you can explore other types of programming languages. Focus on one first to build a strong foundation.
How much time does learning a programming language take?
It depends on the language and your goals. Simple languages like Python might take a few weeks to understand the basics, while complex ones like C++ could take months. Practicing 1-2 hours daily with small projects can help you learn faster.
Do I need a powerful computer to code?
Not usually! Most programming languages, like Python, JavaScript, or SQL, run on basic computers. For game development with C++ or heavy data science tasks, a stronger computer helps, but beginners can start with almost any laptop or PC.
Are free tools enough to start coding?
Yes, absolutely! Free tools like Visual Studio Code, Replit, or online compilers for Python and JavaScript are great for beginners. Many languages also have free tutorials and communities, so you can explore different types of programming languages without spending money.
Can I switch programming languages later?
Definitely! Skills from one language, like problem-solving or logic, transfer to others. For example, learning Python makes it easier to pick up Java or R later. Start with what are the different types of programming languages that match your goals, then switch as needed.
Is coding only for math geniuses?
No way! While some fields like data science use math, many coding tasks, like web development with JavaScript or automation with Python, don't require advanced math. Basic logic and practice are enough to start coding.
Looking to turn your idea into reality?
Don't wait for the "perfect" moment. Pick a language, try it out, and see where it takes you. Whether you're exploring or just want to create something cool, your coding journey starts with that first line of code.
Partner with CISIN for expert guidance and development support. Our skilled developers can help you choose the best tools and bring your vision to life quickly and efficiently. Let's build something great together!